一 概述
- shared_preferences,它保存数据的形式为 Key-Value(键值对),支持 Android 和 iOS
- shared_preferences 是一个第三方插件,在 Android 中使用
SharedPreferences
,在 iOS中使用 NSUserDefaults
二 添加依赖
2.1 依赖地址
- pub 地址:https://pub.flutter-io.cn/packages/shared_preferences
- Github 地址:https://github.com/flutter/plugins/tree/master/packages/shared_preferences/shared_preferences
2.2 添加依赖
在项目的 pubspec.yaml
文件中添加依赖
1 2
| dependencies: shared_preferences: ^2.0.6
|
执行命令
三 shared_preferences操作
3.1 支持数据类型
shared_preferences 支持的数据类型有 int、double、bool、string、stringList
3.2 shared_preferences实例化
同步操作
1
| Future<SharedPreferences> _prefs = SharedPreferences.getInstance();
|
异步操作(async)
1
| var prefs = await SharedPreferences.getInstance();
|
3.3 数据的基本操作(int为例)
3.3.1 读取数据
1 2 3 4 5
| Future<int> _readData() async { var prefs = await SharedPreferences.getInstance(); var result = prefs.getInt('Key_Int'); return result ?? 0; }
|
3.3.2 保存数据
1 2 3 4
| _saveData() async { var prefs = await SharedPreferences.getInstance(); prefs.setInt('Key_Int', 12); }
|
3.3.3 删除数据
1 2 3 4
| Future<bool> _deleteData() async { var prefs = await SharedPreferences.getInstance(); prefs.remove('Key'); }
|
3.3.4 清除所有数据
1 2 3 4
| Future<bool> _clearData() async { var prefs = await SharedPreferences.getInstance(); prefs.clear(); }
|
3.4 Key 相关操作
3.4.1 获取所有的 Key
1 2 3 4 5
| Future<Set<String>> _getKeys() async { var prefs = await SharedPreferences.getInstance(); var keys = prefs.getKeys(); return keys ?? []; }
|
3.4.2 检测是否 Key 是否存在
1 2 3 4
| Future<bool> _containsKey() async { var prefs = await SharedPreferences.getInstance(); return prefs.containsKey('Key') ?? false; }
|
四 示例
4.1 代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| Future<SharedPreferences> _prefs = SharedPreferences.getInstance(); //sharepreference初始化 late Future<int> _counter;//变量
//依次+1 Future<void> _incrementCounter() async { final SharedPreferences prefs = await _prefs; final int counter = (prefs.getInt('counter') ?? 0) + 1; setState(() { _counter = prefs.setInt("counter", counter).then((bool success) { return counter; }); }); } @override void initState() { super.initState(); _counter = _prefs.then((SharedPreferences prefs) { return (prefs.getInt('counter') ?? 0); }); } body: Center( child: FutureBuilder<int>( future: _counter, builder: (BuildContext context, AsyncSnapshot<int> snapshot) { switch (snapshot.connectionState) { case ConnectionState.waiting: return const CircularProgressIndicator(); default: if (snapshot.hasError) { return Text('Error: ${snapshot.error}'); } else { return Text( 'Button 点击了 ${snapshot.data}次', ); } } })), floatingActionButton: FloatingActionButton( onPressed: _incrementCounter, tooltip: 'Increment', child: const Icon(Icons.add), ),
|
4.2 效果图
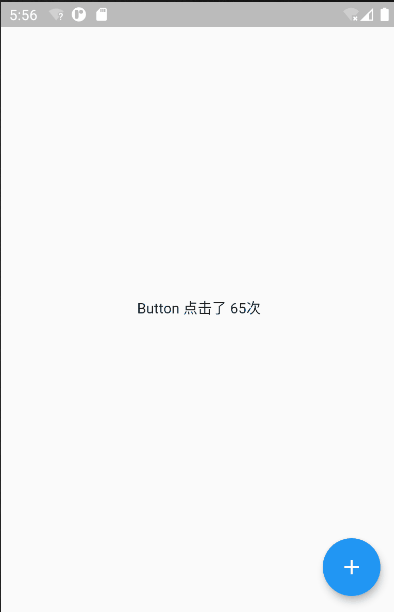
4.3 查看shared_preferences文件